Introduction
Mobile app monetization has evolved significantly over the years, with in-app purchases (IAP) becoming one of the most effective strategies for generating revenue. For Flutter developers, implementing a robust and user-friendly payment system is crucial for app success. This guide will walk you through the process of integrating in-app purchases in your Flutter applications, from initial setup to deployment.
Flutter’s cross-platform capabilities make it an excellent choice for developers looking to reach users across different platforms with a single codebase. By implementing in-app purchases correctly, you can create additional revenue streams while enhancing the user experience with premium content and features.
Why Implement In-App Purchases?
Before diving into the implementation details, let’s understand the benefits of adding in-app purchases to your Flutter application:
- Monetization Strategy: Transform free apps into revenue-generating products by offering premium features
- User Experience: Provide users with the option to enhance their experience through additional content
- Recurring Revenue: Implement subscription models for stable, predictable income
- Platform Support: Flutter’s in-app purchase plugin supports both iOS and Android platforms
- Flexible Offerings: Sell consumable items, non-consumable features, or subscription services
Types of In-App Purchases
There are three main types of in-app purchases you can implement:
- Consumable Products: One-time use items that can be purchased repeatedly (e.g., virtual currency, extra lives)
- Non-Consumable Products: Permanent features that are purchased once (e.g., ad removal, premium features)
- Subscriptions: Recurring payments for continued access to content or services (e.g., monthly premium membership)
Setting Up Your Flutter Environment
Prerequisites
Before implementing in-app purchases, ensure you have:
- Flutter SDK (latest version recommended)
- Developer accounts on Google Play Console and/or Apple App Store Connect
- Basic understanding of Flutter development
Adding the In-App Purchase Plugin
Start by adding the in-app purchase plugin to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
in_app_purchase: ^3.1.5
shared_preferences: ^2.1.1 # For storing purchase state
Run flutter pub get
to install the dependencies.
Configuring Platform-Specific Settings
Android Configuration
In your android/app/build.gradle
file, ensure you have the billing permission:
android {
defaultConfig {
// Other configurations...
minSdkVersion 21 // Required for billing
}
}
Add the billing permission to your AndroidManifest.xml
:
<uses-permission android:name="com.android.vending.BILLING" />
iOS Configuration
For iOS, add the StoreKit framework to your app. In your ios/Runner.xcodeproj/project.pbxproj
file, ensure you have:
frameworks = [
"StoreKit",
// Other frameworks...
];
Implementing In-App Purchases
Initializing the Plugin
First, initialize the in-app purchase plugin in your app:
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:in_app_purchase/in_app_purchase.dart';
class PaymentService {
final InAppPurchase _inAppPurchase = InAppPurchase.instance;
StreamSubscription<List<PurchaseDetails>>? _subscription;
Future<void> initialize() async {
final available = await _inAppPurchase.isAvailable();
if (!available) {
// Store is not available
print('Store is not available');
return;
}
// Set up the subscription to listen for purchase updates
_subscription = _inAppPurchase.purchaseStream.listen(
_onPurchaseUpdate,
onDone: _updateStreamOnDone,
onError: _updateStreamOnError,
);
}
void _onPurchaseUpdate(List<PurchaseDetails> purchaseDetailsList) {
// We'll implement this method later
}
void _updateStreamOnDone() {
_subscription?.cancel();
}
void _updateStreamOnError(dynamic error) {
// Handle errors here
print('Error in purchase stream: $error');
}
void dispose() {
_subscription?.cancel();
}
}
Fetching Available Products
Create a method to load products from the store:
Future<List<ProductDetails>> loadProducts() async {
// Define your product IDs
final Set<String> productIds = {
'premium_upgrade',
'coins_100',
'monthly_subscription'
};
// Query the store for product details
final ProductDetailsResponse response =
await _inAppPurchase.queryProductDetails(productIds);
if (response.error != null) {
print('Error loading products: ${response.error}');
return [];
}
return response.productDetails;
}
Displaying Products to Users
Create a widget to display available products:
class StoreScreen extends StatefulWidget {
@override
_StoreScreenState createState() => _StoreScreenState();
}
class _StoreScreenState extends State<StoreScreen> {
final PaymentService _paymentService = PaymentService();
List<ProductDetails> _products = [];
bool _isLoading = true;
@override
void initState() {
super.initState();
_initializeStore();
}
Future<void> _initializeStore() async {
await _paymentService.initialize();
final products = await _paymentService.loadProducts();
setState(() {
_products = products;
_isLoading = false;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Store')),
body: _isLoading
? Center(child: CircularProgressIndicator())
: ListView.builder(
itemCount: _products.length,
itemBuilder: (context, index) {
final product = _products[index];
return ListTile(
title: Text(product.title),
subtitle: Text(product.description),
trailing: TextButton(
child: Text('${product.price}'),
onPressed: () => _purchaseProduct(product),
),
);
},
),
);
}
Future<void> _purchaseProduct(ProductDetails product) async {
// We'll implement this method next
}
@override
void dispose() {
_paymentService.dispose();
super.dispose();
}
}
Processing Purchases
Add the purchase functionality to your PaymentService:
Future<void> purchaseProduct(ProductDetails product) async {
final PurchaseParam purchaseParam = PurchaseParam(productDetails: product);
if (_isConsumable(product.id)) {
return _inAppPurchase.buyConsumable(purchaseParam: purchaseParam);
} else {
return _inAppPurchase.buyNonConsumable(purchaseParam: purchaseParam);
}
}
bool _isConsumable(String productId) {
// Define which products are consumable
final consumables = {'coins_100'};
return consumables.contains(productId);
}
Now, implement the purchase handling in the StoreScreen:
Future<void> _purchaseProduct(ProductDetails product) async {
try {
await _paymentService.purchaseProduct(product);
// Purchase initiated - results will come through the purchase stream
} catch (e) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('Failed to initiate purchase: $e')),
);
}
}
Handling Purchase Updates
Now, let’s implement the _onPurchaseUpdate
method in the PaymentService:
void _onPurchaseUpdate(List<PurchaseDetails> purchaseDetailsList) {
for (final purchaseDetails in purchaseDetailsList) {
if (purchaseDetails.status == PurchaseStatus.pending) {
// Show loading UI
_showPendingUI();
} else if (purchaseDetails.status == PurchaseStatus.error) {
// Handle error
_handleError(purchaseDetails.error!);
} else if (purchaseDetails.status == PurchaseStatus.purchased ||
purchaseDetails.status == PurchaseStatus.restored) {
// Verify purchase on server and deliver product
_verifyAndDeliverProduct(purchaseDetails);
}
if (purchaseDetails.pendingCompletePurchase) {
// Complete the purchase to finish the transaction
_inAppPurchase.completePurchase(purchaseDetails);
}
}
}
void _showPendingUI() {
// Show loading indicator or pending message
print('Purchase pending...');
}
void _handleError(IAPError error) {
// Handle the error appropriately
print('Purchase error: ${error.message}');
}
Future<void> _verifyAndDeliverProduct(PurchaseDetails purchase) async {
// 1. Verify the purchase with your server (recommended)
final bool isValid = await _verifyPurchase(purchase);
if (!isValid) {
// Purchase verification failed
print('Purchase verification failed');
return;
}
// 2. Deliver the product based on the productID
switch (purchase.productID) {
case 'premium_upgrade':
await _unlockPremiumFeatures();
break;
case 'coins_100':
await _addCoins(100);
break;
case 'monthly_subscription':
await _activateSubscription(purchase.purchaseID!, 30); // 30 days
break;
default:
print('Unknown product: ${purchase.productID}');
}
// 3. Notify listeners about successful purchase
print('Purchase completed: ${purchase.productID}');
}
Future<bool> _verifyPurchase(PurchaseDetails purchase) async {
// In a real app, you would verify the purchase with your backend
// This is just a placeholder that always returns true
return true;
}
// Product delivery methods
Future<void> _unlockPremiumFeatures() async {
// Store premium status in local storage
// SharedPreferences prefs = await SharedPreferences.getInstance();
// prefs.setBool('isPremium', true);
}
Future<void> _addCoins(int amount) async {
// Update coin balance in storage
// SharedPreferences prefs = await SharedPreferences.getInstance();
// int currentCoins = prefs.getInt('coins') ?? 0;
// prefs.setInt('coins', currentCoins + amount);
}
Future<void> _activateSubscription(String purchaseId, int days) async {
// Calculate expiration date
final DateTime now = DateTime.now();
final DateTime expiryDate = now.add(Duration(days: days));
// Store subscription info
// SharedPreferences prefs = await SharedPreferences.getInstance();
// prefs.setString('subscriptionId', purchaseId);
// prefs.setInt('subscriptionExpiry', expiryDate.millisecondsSinceEpoch);
}
Server-Side Purchase Verification
For security, it’s essential to verify purchases on your server. Here’s a simplified approach:
- Send the purchase receipt to your server
- From your server, validate the receipt with Google Play API or Apple’s App Store API
- Store the validation result and grant the purchased content only after verification
Future<bool> _verifyPurchaseWithServer(PurchaseDetails purchase) async {
// This would be an HTTP call to your backend
try {
final response = await http.post(
Uri.parse('https://your-backend.com/verify-purchase'),
body: {
'productId': purchase.productID,
'purchaseToken': purchase.verificationData.serverVerificationData,
'platform': Platform.isAndroid ? 'android' : 'ios',
},
);
if (response.statusCode == 200) {
final data = jsonDecode(response.body);
return data['isValid'] == true;
}
} catch (e) {
print('Error verifying purchase with server: $e');
}
return false;
}
Testing In-App Purchases
Testing on Android
- Create a testing account in Google Play Console
- Add test users in the app’s Testing track
- Use Google’s test payment methods for simulating purchases
Testing on iOS
- Create sandbox testers in App Store Connect
- Use these sandbox accounts to test purchases without real charges
- Verify receipt validation in the sandbox environment
Platform-Specific Setup
Google Play Console Setup
- Log in to Google Play Console
- Navigate to your app > Monetize > Products
- Create in-app products with the same product IDs used in your code
- Set prices and descriptions for each product
- Publish your products to testing
App Store Connect Setup
- Log in to App Store Connect
- Navigate to your app > Features > In-App Purchases
- Create new in-app purchases
- Set product details and pricing
- Submit for review
Best Practices
- Always verify purchases server-side to prevent fraudulent transactions
- Implement restore purchases functionality for users who reinstall your app
- Handle subscription management appropriately, including renewals and cancellations
- Store purchase state securely, preferably with server validation
- Test thoroughly across different devices and platforms
- Implement analytics to track purchase conversions and user behavior
- Provide clear descriptions of what users receive with each purchase
Handling Edge Cases
Interrupted Purchases
If a purchase is interrupted (e.g., due to network issues), the purchase stream will notify your app when it resumes. Make sure your app can handle these cases.
Restoring Purchases
Implement a “Restore Purchases” button for users who have previously purchased non-consumable items:
Future<void> restorePurchases() async {
await _inAppPurchase.restorePurchases();
// The purchase stream will receive the restored purchases
}
Handling Subscriptions
For subscription products, you need to handle renewal states and expiration:
bool isSubscriptionActive() {
// Check if the current time is before the expiration date
SharedPreferences prefs = await SharedPreferences.getInstance();
final expiryTimestamp = prefs.getInt('subscriptionExpiry') ?? 0;
if (expiryTimestamp == 0) return false;
final expiryDate = DateTime.fromMillisecondsSinceEpoch(expiryTimestamp);
return DateTime.now().isBefore(expiryDate);
}
Conclusion
Implementing in-app purchases in Flutter applications opens up new revenue opportunities while enhancing the user experience with premium content and features. By following this guide, you’ve learned how to:
- Set up the in-app purchase plugin
- Configure platform-specific settings
- Fetch and display product information
- Process purchases and deliver content
- Verify purchases securely
- Handle various edge cases
Remember that a successful in-app purchase implementation requires both technical excellence and a well-thought-out monetization strategy. Consider your users’ needs and preferences when designing your premium offerings, and always provide clear value for the purchases you offer.
With the knowledge gained from this guide, you’re well-equipped to implement a robust in-app purchase system in your Flutter applications. Happy coding!
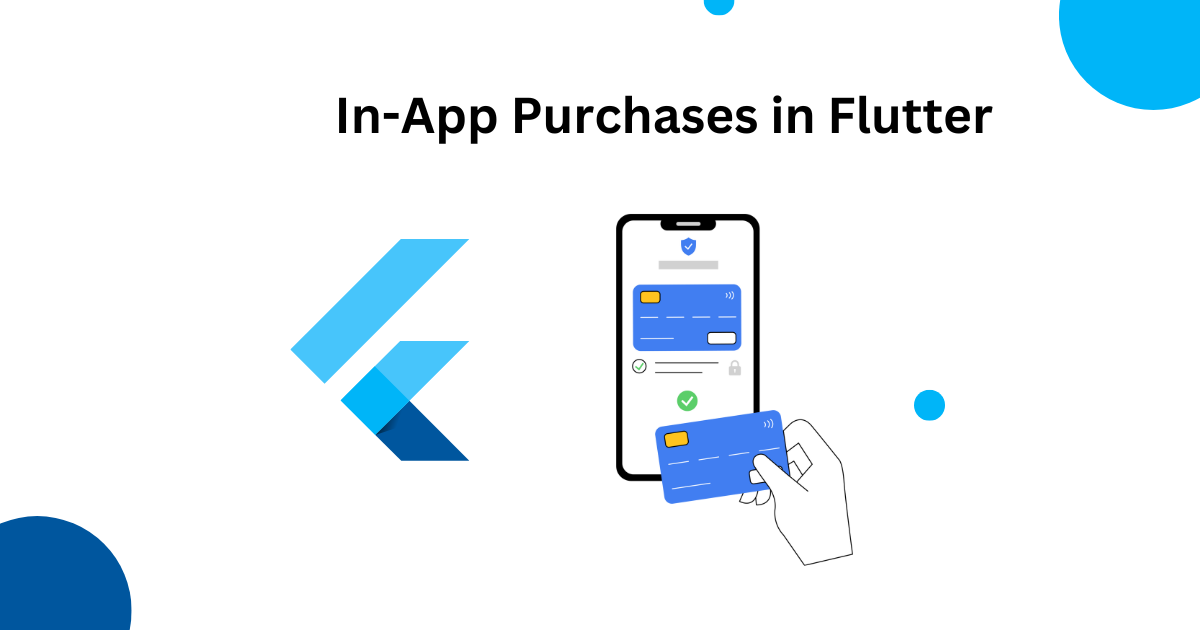
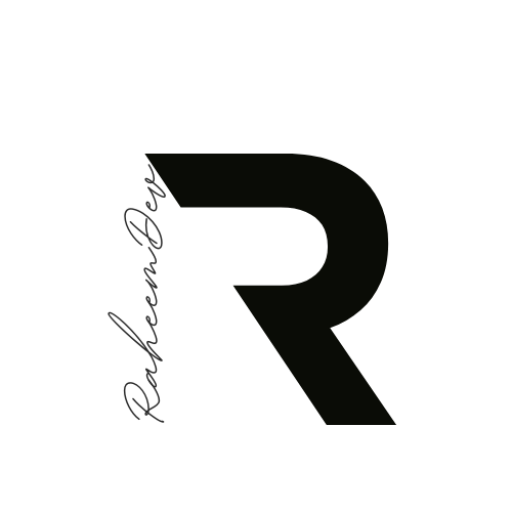