1. Introduction
In the world of modern app development, responsiveness is key. Users expect smooth, uninterrupted experiences, even when apps are performing complex operations behind the scenes. This is where Futures in Flutter come into play.
Futures represent values or errors that are not yet available but will be at some point in the future. They are a cornerstone of asynchronous programming in Dart, allowing developers to write non-blocking code that can handle time-consuming tasks without freezing the user interface.
In Flutter, Google’s UI toolkit for building natively compiled applications, Futures are indispensable. They enable developers to create fluid, responsive apps that can handle network requests, file operations, and other potentially slow processes without compromising the user experience.
2. Basic Concepts
To understand Futures, we need to grasp a few fundamental concepts:
Asynchronous Programming
Asynchronous programming is a technique that enables your program to start a potentially long-running task and still be able to respond to other events while that task runs, rather than having to wait until that task has finished.
The Event Loop in Dart
Dart uses an event loop to handle asynchronous operations. The event loop continuously checks for and dispatches events or messages in a program. When an asynchronous operation completes, it adds an event to the queue, which the event loop then processes.
Difference Between Synchronous and Asynchronous Code
Synchronous code runs in sequence – each statement waits for the previous one to finish before executing. Asynchronous code, on the other hand, allows multiple operations to proceed concurrently. While one operation is waiting (e.g., for a network response), other operations can continue to execute.
3. Creating and Using Futures
How to Create a Future
In Dart, you can create a Future in several ways:
// Using Future.delayed
Future<String> fetchUserOrder() {
return Future.delayed(Duration(seconds: 2), () => 'Large Latte');
}
// Using async/await
Future<String> fetchUserOrder() async {
await Future.delayed(Duration(seconds: 2));
return 'Large Latte';
}
Using async
and await
The async
and await
keywords provide a clean syntax for working with Futures:
void main() async {
print('Fetching user order...');
String order = await fetchUserOrder();
print('Your order is: $order');
}
Handling Errors with try-catch
Error handling is crucial when working with Futures. The try-catch
block allows you to gracefully handle potential errors:
try {
String order = await fetchUserOrder();
print('Your order is: $order');
} catch (error) {
print('Failed to fetch order: $error');
}
4. Common Use Cases
Futures are versatile and find applications in various scenarios:
4.1. Network Requests
Futures are ideal for handling HTTP requests, which are inherently asynchronous:
Future<void> fetchData() async {
final response = await http.get('https://api.example.com/data');
if (response.statusCode == 200) {
print('Data received: ${response.body}');
} else {
throw Exception('Failed to load data');
}
}
4.2. File I/O Operations
Reading from or writing to files can be time-consuming. Futures make these operations non-blocking:
Future<String> readFile(String path) async {
final file = File(path);
return await file.readAsString();
}
4.3. Database Operations
When working with databases, operations like querying or updating can take time. Futures ensure these operations don’t freeze the UI:
Future<List<User>> fetchUsers() async {
final db = await database;
final List<Map<String, dynamic>> maps = await db.query('users');
return List.generate(maps.length, (i) => User.fromMap(maps[i]));
}
4.4. Delayed Computations
For operations that need to be delayed, Futures provide a straightforward solution:
Future<void> delayedGreeting(String name) async {
await Future.delayed(Duration(seconds: 2));
print('Hello, $name!');
}
4.5. Parallel Computations
Futures allow you to run multiple computations in parallel and wait for all of them to complete:
Future<void> fetchMultipleUsers() async {
final futures = [fetchUser(1), fetchUser(2), fetchUser(3)];
final users = await Future.wait(futures);
users.forEach(print);
}
5. Advanced Concepts
Chaining Futures
Futures can be chained together, allowing you to perform a sequence of asynchronous operations:
void fetchUserAndOrders() {
fetchUser()
.then((user) => fetchOrders(user.id))
.then((orders) => print('User has ${orders.length} orders'))
.catchError((error) => print('Error: $error'));
}
Working with Multiple Futures
Future.wait
allows you to work with multiple Futures concurrently:
Future<void> fetchUserData() async {
final results = await Future.wait([
fetchUserProfile(),
fetchUserPosts(),
fetchUserFriends()
]);
final profile = results[0];
final posts = results[1];
final friends = results[2];
// Use the data...
}
Timeouts and Cancellation
You can add timeouts to Futures to prevent them from hanging indefinitely:
Future<String> fetchWithTimeout() async {
return await fetchData().timeout(
Duration(seconds: 5),
onTimeout: () => throw TimeoutException('The request took too long.'),
);
}
6. Best Practices and Pitfalls
Error Handling Strategies
- Always use
try-catch
blocks or.catchError()
to handle potential errors. - Consider using
Future.value()
orFuture.error()
for immediate Future resolution.
Avoiding Common Mistakes
- Don’t forget to await Futures or use
.then()
to handle their results. - Avoid nesting Futures deeply; instead, use
async/await
or Future chaining.
Performance Considerations
- Use
compute()
function for CPU-intensive tasks to avoid blocking the main isolate. - Consider caching results of expensive Future operations.
7. Comparison with Other Languages
Futures in Dart are similar to Promises in JavaScript or Tasks in C#. However, Dart’s async/await
syntax makes working with Futures particularly clean and intuitive. Unlike JavaScript, Dart is a strongly typed language, which provides additional safety when working with asynchronous code.
8. Conclusion
Futures are a powerful feature in Dart and Flutter, enabling developers to write efficient, non-blocking code. They are essential for creating responsive applications that can handle complex operations without compromising user experience.
As asynchronous programming continues to be crucial in modern app development, mastering Futures will remain an invaluable skill for Dart and Flutter developers. Keep exploring, experimenting, and building with Futures to create smoother, more efficient applications.
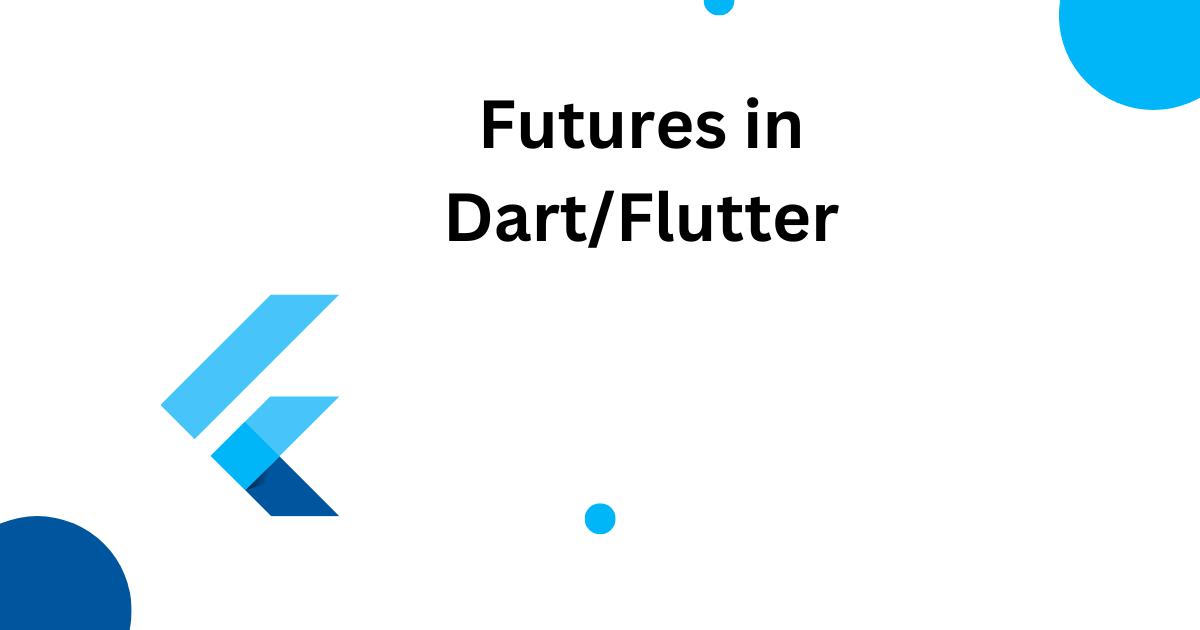
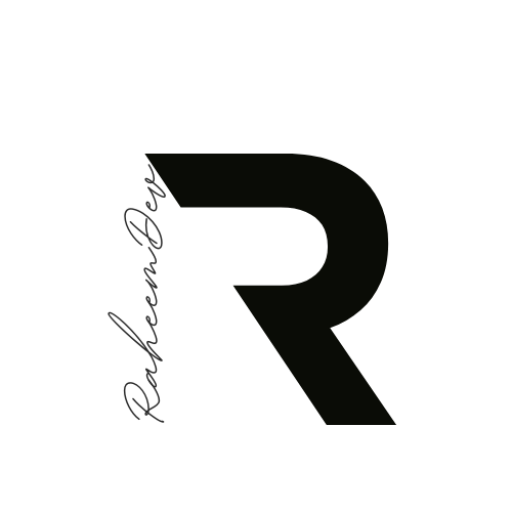