Introduction
The IntrinsicHeight widget in Flutter is a powerful layout tool that forces its children to have the same height based on the maximum intrinsic height among them. While it can be performance-intensive for complex layouts, it solves specific layout challenges that are difficult to handle with other widgets.
Understanding IntrinsicHeight
The IntrinsicHeight widget traverses its children to determine the maximum intrinsic height and then forces all children to adopt that height. This is particularly useful when:
- You need rows of widgets to have equal heights
- You want to size widgets based on their content
- You need to align widgets with varying content lengths
Basic Example
Here’s a simple example showing how IntrinsicHeight ensures equal height for different widgets:
import 'package:flutter/material.dart';
class IntrinsicHeightDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('IntrinsicHeight Demo'),
),
body: Center(
child: IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Container(
width: 100,
color: Colors.blue,
child: Text('ShortnText'),
),
Container(
width: 100,
color: Colors.green,
child: Text('MuchnLongernTextnContent'),
),
Container(
width: 100,
color: Colors.red,
child: Text('MediumnLengthnText'),
),
],
),
),
),
);
}
}
Practical Use Cases
1. Card Layout with Varying Content
class CardLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: EdgeInsets.all(16.0),
child: IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Expanded(
child: Card(
child: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
Icon(Icons.star, size: 48),
Text('Basic Plan'),
Text('\$9.99/month'),
],
),
),
),
),
SizedBox(width: 16),
Expanded(
child: Card(
child: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
Icon(Icons.star, size: 48),
Text('Premium Plan'),
Text('\$19.99/month'),
Text('Includes additional features'),
Text('24/7 Support'),
],
),
),
),
),
],
),
),
),
);
}
}
2. Form Layout with Labels and Inputs
class FormLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 100,
child: Text('Name:', style: TextStyle(fontSize: 16)),
),
Expanded(
child: TextField(
decoration: InputDecoration(
border: OutlineInputBorder(),
),
),
),
],
),
),
SizedBox(height: 16),
IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 100,
child: Text('Address:', style: TextStyle(fontSize: 16)),
),
Expanded(
child: TextField(
maxLines: 3,
decoration: InputDecoration(
border: OutlineInputBorder(),
),
),
),
],
),
),
],
),
),
);
}
}
Best Practices and Performance Considerations
- Limited Usage
- Use IntrinsicHeight only when necessary, as it can be computationally expensive
- Consider alternatives like ConstrainedBox or SizedBox when possible
- Avoid Nesting
- Don’t nest multiple IntrinsicHeight widgets
- Keep the widget tree shallow to maintain performance
- Performance Optimization
- Use const constructors where possible
- Consider caching widgets that don’t need to rebuild
- Limit the number of children
Common Pitfalls
- Infinite Height
// DON'T DO THIS IntrinsicHeight( child: ListView( // ListView has infinite height children: [...], ), )
- Unnecessary Usage
// UNNECESSARY - Use SizedBox instead IntrinsicHeight( child: Container( height: 100, child: Text('Fixed height content'), ), )
Alternatives to Consider
- ConstrainedBox: When you need to enforce specific size constraints
- SizedBox: For fixed dimensions
- Container: When you need simple size control
- AspectRatio: For maintaining specific width-to-height ratios
Conclusion
IntrinsicHeight is a powerful widget for ensuring consistent heights across multiple widgets, particularly useful in scenarios where content sizes are dynamic or unknown. While it should be used judiciously due to performance considerations, it solves specific layout challenges that would be difficult to address otherwise.
Remember to always consider the performance implications and whether simpler alternatives might suffice for your specific use case. When used appropriately, IntrinsicHeight can significantly improve the visual consistency of your Flutter applications.

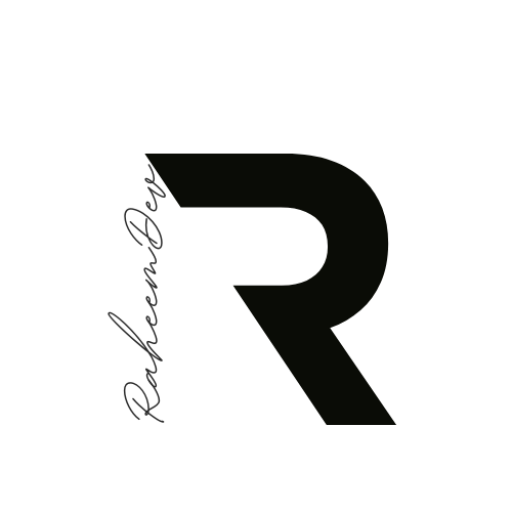