What is Flow Builder?
Flow Builder is a Flutter package that helps manage multi-step flows in your application. It’s designed to simplify the process of creating and maintaining sequences of screens or forms, such as user registration, checkout processes, or complex setup wizards.Why Use Flow Builder?
- Simplified State Management: Flow Builder handles the state of your multi-step process, reducing boilerplate code.
- Easy Navigation: It manages navigation between steps, including back navigation, with minimal effort.
- Flexible and Customizable: Adapt Flow Builder to fit various types of multi-step processes in your app.
- Improved Code Organization: Keep your flow logic separate from your UI code for better maintainability.
How to Implement Flow Builder
Let’s walk through a basic implementation of Flow Builder using a user registration flow as an example.Step 1: Add the Dependency
First, add Flow Builder to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flow_builder: ^0.1.0
Step 2: Create Your Flow State
Define a class to hold the state of your flow:class RegistrationData {
final String? name;
final String? email;
final bool personalInfoCompleted;
final bool contactInfoCompleted;
RegistrationData({
this.name,
this.email,
this.personalInfoCompleted = false,
this.contactInfoCompleted = false,
});
RegistrationData copyWith({
String? name,
String? email,
bool? personalInfoCompleted,
bool? contactInfoCompleted,
}) {
return RegistrationData(
name: name ?? this.name,
email: email ?? this.email,
personalInfoCompleted: personalInfoCompleted ?? this.personalInfoCompleted,
contactInfoCompleted: contactInfoCompleted ?? this.contactInfoCompleted,
);
}
}
Step 3: Implement the Flow
UseFlowBuilder
to manage your registration flow:
class RegistrationFlow extends StatelessWidget {
@override
Widget build(BuildContext context) {
return FlowBuilder<RegistrationData>(
state: RegistrationData(),
onGeneratePages: (RegistrationData data, List<Page> pages) {
return [
MaterialPage(child: PersonalInfoStep()),
if (data.personalInfoCompleted)
MaterialPage(child: ContactInfoStep()),
if (data.contactInfoCompleted)
MaterialPage(child: AccountCreationStep()),
];
},
);
}
}
Step 4: Create Individual Steps
Implement each step of your flow as a separate widget:class PersonalInfoStep extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Personal Information')),
body: Center(
child: ElevatedButton(
child: Text('Next'),
onPressed: () {
context.flow<RegistrationData>().update((data) =>
data.copyWith(name: 'John Doe', personalInfoCompleted: true));
},
),
),
);
}
}
class ContactInfoStep extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Contact Information')),
body: Center(
child: ElevatedButton(
child: Text('Next'),
onPressed: () {
context.flow<RegistrationData>().update((data) =>
data.copyWith(email: 'john@gmail.com', contactInfoCompleted: true));
},
),
),
);
}
}
class AccountCreationStep extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Account Creation')),
body: Center(
child: ElevatedButton(
child: Text('Create Account'),
onPressed: () {
// Here you would typically send the data to your backend
final data = context.flow<RegistrationData>().state;
print('Registration complete: ${data.name}, ${data.email}');
// Navigate to home screen or show success message
},
),
),
);
}
}
Best Practices
- Keep It Simple: Start with a basic flow and add complexity as needed.
- Validate Each Step: Ensure data is valid before moving to the next step.
- Handle Back Navigation: Consider how users can edit previous steps.
- Use Meaningful Names: Choose clear names for your flow states and steps.
Conclusion
Flutter Flow Builder is a game-changer for Flutter developers dealing with multi-step processes. By simplifying state management and navigation, it allows you to focus on creating a great user experience. Whether you’re building a complex onboarding process or a simple two-step form, Flow Builder can help streamline your development process. Give Flow Builder a try in your next Flutter project and experience the difference it can make in managing your app’s flows!Thank you for reading 👋
I hope you enjoyed this article. If you have any queries or suggestions please let me know in the comments down below.
I’m Shehzad Raheem 📱 Flutter Developer and I help firms to fulfill their Mobile Application Development, Android Development, and Flutter Development needs. If you want to discuss any project, drop me a message
Summary
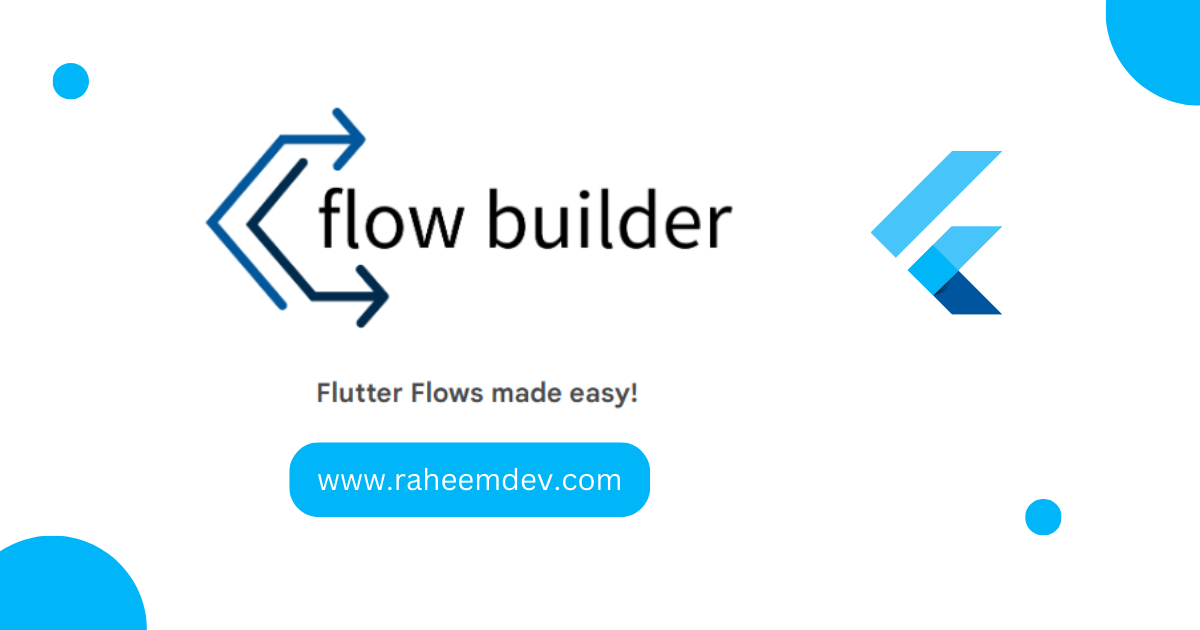
Article Name
Flutter Flow Builder: Simplifying Multi-Step Processes
Description
Flow Builder simplifies managing multi-step flows in Flutter apps, perfect for user registration, checkout processes, or setup wizards.
Author
Shehzad Raheem
Publisher Name
raheemdev.com
Publisher Logo
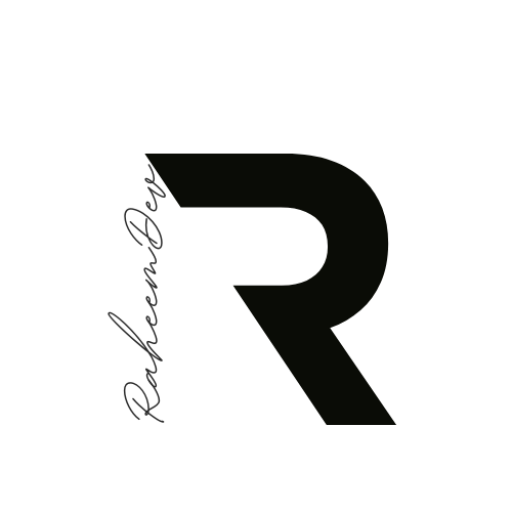