In this comprehensive guide, we’ll walk through the process of implementing email verification in Flutter using Twilio Verify. We’ll cover the setup of Twilio Verify for email, followed by a complete Flutter implementation.
Here you can findĀ Twilio Phone Verification in Flutter
Setting up Twilio Verify for Email
Before we dive into the Flutter code, it’s crucial to set up Twilio Verify for email. Follow these steps based on the Twilio documentation: Now that we have set up Twilio Verify for email, let’s implement the Flutter application.
Flutter Implementation
Prerequisites
Before we begin, ensure you have:
- A Flutter development environment set up
- The
twilio_phone_verify
package (version 2.0.0 or later) added to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
twilio_phone_verify: ^2.0.0
Full Implementation
Let’s break down the entire implementation, explaining each part in detail:
- Main App Structure
import 'package:flutter/material.dart';
import 'package:twilio_phone_verify/twilio_phone_verify.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Twilio Email Verify',
theme: ThemeData(
primaryColor: Color(0xFF233659),
),
home: EmailVerification(),
);
}
}
This sets up the main app structure, importing necessary packages and defining the MyApp
widget.
- Email Verification State Management
enum VerificationState { enterEmail, enterVerificationCode }
class EmailVerification extends StatefulWidget {
@override
_EmailVerificationState createState() => _EmailVerificationState();
}
class _EmailVerificationState extends State<EmailVerification> {
TwilioPhoneVerify _twilioVerify;
var verificationState = VerificationState.enterEmail;
var emailController = TextEditingController();
var verificationCodeController = TextEditingController();
bool loading = false;
String errorMessage;
String successMessage;
@override
void initState() {
super.initState();
_twilioVerify = TwilioPhoneVerify(
accountSid: 'YOUR_ACCOUNT_SID',
serviceSid: 'YOUR_SERVICE_SID',
authToken: 'YOUR_AUTH_TOKEN'
);
}
This section manages the state for email verification, including controllers for input fields and a TwilioPhoneVerify
instance.
- Build Method and State Management
@override
Widget build(BuildContext context) {
return verificationState == VerificationState.enterEmail
? _buildEnterEmail()
: _buildEnterVerificationCode();
}
void changeErrorMessage(String message) =>
setState(() => errorMessage = message);
void changeSuccessMessage(String message) =>
setState(() => successMessage = message);
void changeLoading(bool status) => setState(() => loading = status);
void switchToVerificationCode() {
changeSuccessMessage(null);
changeErrorMessage(null);
changeLoading(false);
setState(() {
verificationState = VerificationState.enterVerificationCode;
});
}
void switchToEmail() {
if (loading) return;
changeSuccessMessage(null);
changeErrorMessage(null);
setState(() {
verificationState = VerificationState.enterEmail;
});
}
These methods handle state changes and determine which screen to display.
- Sending Verification Code
void sendCode() async {
if (emailController.text.isEmpty || loading) return;
changeLoading(true);
TwilioResponse twilioResponse = await _twilioVerify.sendEmailCode(
emailController.text,
channelConfiguration: EmailChannelConfiguration(
from: "verify@yourdomain.com",
from_name: "Your App Name",
template_id: "YOUR_SENDGRID_TEMPLATE_ID",
usernameSubstitution: emailController.text.split('@')[0]
)
);
if (twilioResponse.successful) {
changeSuccessMessage('Verification code sent to ${emailController.text}');
await Future.delayed(Duration(seconds: 1));
switchToVerificationCode();
} else {
changeErrorMessage(twilioResponse.errorMessage);
}
changeLoading(false);
}
This method sends the verification code to the entered email address using Twilio’s API.
- Verifying Email Code
void verifyCode() async {
if (emailController.text.isEmpty ||
verificationCodeController.text.isEmpty ||
loading) return;
changeLoading(true);
TwilioResponse twilioResponse = await _twilioVerify.verifyEmailCode(
email: emailController.text,
code: verificationCodeController.text
);
if (twilioResponse.successful) {
if (twilioResponse.verification.status == VerificationStatus.approved) {
changeSuccessMessage('Email is verified');
} else {
changeSuccessMessage('Invalid code');
}
} else {
changeErrorMessage(twilioResponse.errorMessage);
}
changeLoading(false);
}
This method verifies the email code entered by the user.
- UI for Email Input
Widget _buildEnterEmail() {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(40.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: emailController,
keyboardType: TextInputType.emailAddress,
decoration: InputDecoration(labelText: 'Enter Email Address'),
),
SizedBox(height: 20),
Container(
width: double.infinity,
height: 40,
child: ElevatedButton(
onPressed: sendCode,
style: ElevatedButton.styleFrom(
primary: Theme.of(context).primaryColor,
),
child: loading
? _loader()
: Text(
'Send Verification Code',
style: TextStyle(color: Colors.white),
),
),
),
if (errorMessage != null) ...[
SizedBox(height: 30),
_errorWidget()
],
if (successMessage != null) ...[
SizedBox(height: 30),
_successWidget()
]
],
),
),
);
}
This builds the UI for entering the email address.
- UI for Verification Code Input
Widget _buildEnterVerificationCode() {
return Scaffold(
appBar: AppBar(
elevation: 0,
backgroundColor: Colors.transparent,
leading: IconButton(
icon: Icon(
Icons.arrow_back_ios,
size: 18,
color: Theme.of(context).primaryColor,
),
onPressed: switchToEmail,
),
),
body: Padding(
padding: const EdgeInsets.all(40.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: verificationCodeController,
keyboardType: TextInputType.number,
decoration: InputDecoration(labelText: 'Enter Verification Code'),
),
SizedBox(height: 20),
Container(
width: double.infinity,
height: 40,
child: ElevatedButton(
onPressed: verifyCode,
style: ElevatedButton.styleFrom(
primary: Theme.of(context).primaryColor,
),
child: loading
? _loader()
: Text(
'Verify',
style: TextStyle(color: Colors.white),
),
),
),
if (errorMessage != null) ...[
SizedBox(height: 30),
_errorWidget()
],
if (successMessage != null) ...[
SizedBox(height: 30),
_successWidget()
]
],
),
),
);
}
This builds the UI for entering the verification code.
- Helper Widgets
Widget _loader() => SizedBox(
height: 15,
width: 15,
child: CircularProgressIndicator(
strokeWidth: 2,
valueColor: AlwaysStoppedAnimation(Colors.white),
),
);
Widget _errorWidget() => Material(
borderRadius: BorderRadius.circular(5),
color: Colors.red.withOpacity(.1),
child: Padding(
padding: EdgeInsets.symmetric(vertical: 10, horizontal: 15),
child: Row(
children: [
Expanded(
child: Text(
errorMessage,
style: TextStyle(color: Colors.red),
),
),
IconButton(
icon: Icon(Icons.close, size: 16),
onPressed: () => changeErrorMessage(null),
)
],
),
),
);
Widget _successWidget() => Material(
borderRadius: BorderRadius.circular(5),
color: Colors.green.withOpacity(.1),
child: Padding(
padding: EdgeInsets.symmetric(vertical: 10, horizontal: 15),
child: Row(
children: [
Expanded(
child: Text(
successMessage,
style: TextStyle(color: Colors.green),
),
),
IconButton(
icon: Icon(Icons.close, size: 16),
onPressed: () => changeSuccessMessage(null),
)
],
),
),
);
These helper methods create reusable widgets for the loading indicator, error messages, and success messages.
Conclusion
This comprehensive guide covers the setup of Twilio Verify for email and the entire implementation of email verification in a Flutter app. By following this guide and understanding each component, you can successfully integrate email verification into your Flutter application, enhancing its security and user authentication process.
Remember to handle edge cases, implement proper error handling, and consider additional security measures like rate limiting to create a robust and secure verification system. Always keep your Twilio credentials secure and never expose them in client-side code.
With this implementation, you’re now ready to provide a secure and user-friendly email verification process in your Flutter application!
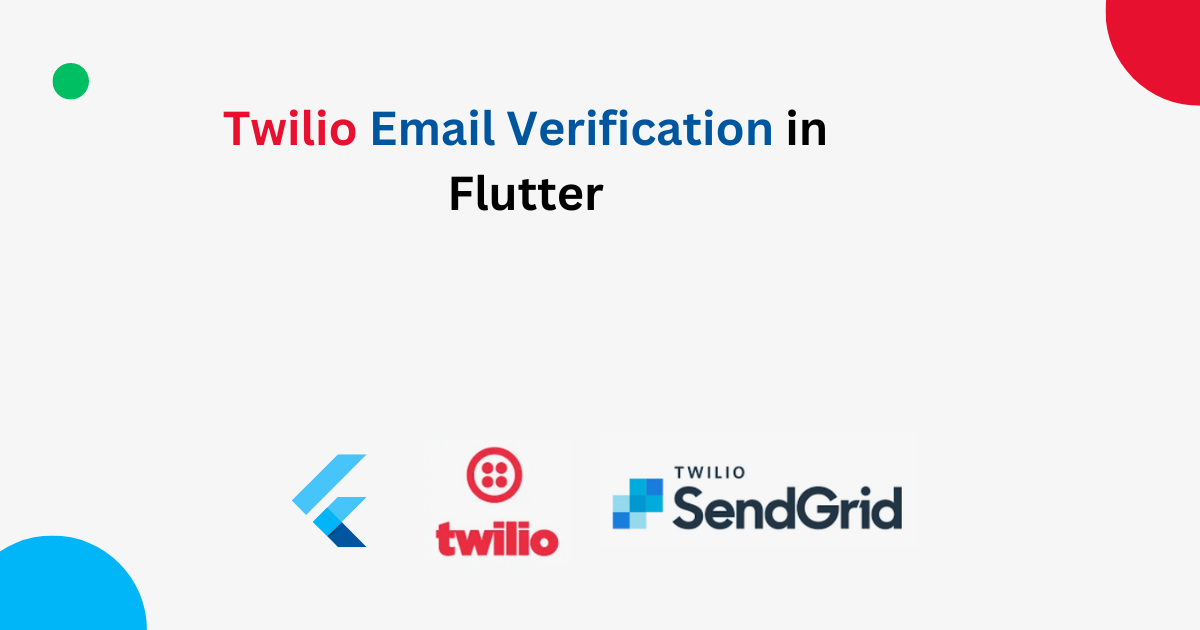
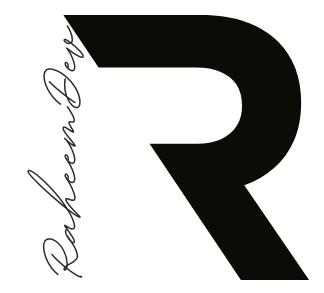