Table of Contents
- Introduction
- What is LayerLink?
- How to Use LayerLink in Flutter
- Benefits of LayerLink
- Practical Example
- Conclusion
Introduction
In the world of Flutter development, creating dynamic and responsive user interfaces often requires precise positioning of widgets relative to others. This is where LayerLink
comes into play, offering a powerful solution for connecting and aligning widgets across different parts of the widget tree.
What is LayerLink?
LayerLink
is a class in Flutter that establishes a connection between two widgets that are not necessarily in the same part of the widget tree. It’s part of the rendering
library and works in conjunction with CompositedTransformTarget
and CompositedTransformFollower
widgets to create a link between two layers in the render tree.
How to Use LayerLink
Using LayerLink
involves three main steps:
- Create a
LayerLink
instance. - Use
CompositedTransformTarget
to mark the reference widget. - Use
CompositedTransformFollower
to position the follower widget relative to the target.
Here’s a basic structure:
final LayerLink _layerLink = LayerLink();
// In your build method:
CompositedTransformTarget(
link: _layerLink,
child: YourReferenceWidget(),
)
// Somewhere else in your widget tree:
CompositedTransformFollower(
link: _layerLink,
offset: Offset(0, 20), // Adjust as needed
child: YourFollowerWidget(),
)
Benefits of LayerLink
- Cross-Tree Positioning: LayerLink allows you to position widgets relative to each other even when they’re in different parts of the widget tree.
- Responsive Design: It helps in creating responsive designs by maintaining relative positioning across different screen sizes and orientations.
- Overlay Management: It’s particularly useful for managing overlays, tooltips, and popups that need to be positioned relative to specific widgets.
- Performance: LayerLink provides an efficient way to update positions without rebuilding the entire widget tree.
- Flexibility: It offers fine-grained control over positioning, allowing for complex UI layouts.
Practical Example
Let’s create a simple tooltip that appears above a button using LayerLink
:
import 'package:flutter/material.dart';
class LayerLinkExample extends StatefulWidget {
@override
_LayerLinkExampleState createState() => _LayerLinkExampleState();
}
class _LayerLinkExampleState extends State<LayerLinkExample> {
final LayerLink _layerLink = LayerLink();
OverlayEntry? _overlayEntry;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('LayerLink Example')),
body: Center(
child: CompositedTransformTarget(
link: _layerLink,
child: ElevatedButton(
child: Text('Show Tooltip'),
onPressed: _toggleTooltip,
),
),
),
);
}
void _toggleTooltip() {
if (_overlayEntry == null) {
_overlayEntry = _createOverlayEntry();
Overlay.of(context)?.insert(_overlayEntry!);
} else {
_overlayEntry?.remove();
_overlayEntry = null;
}
}
OverlayEntry _createOverlayEntry() {
return OverlayEntry(
builder: (context) => Positioned(
width: 200,
child: CompositedTransformFollower(
link: _layerLink,
offset: Offset(0, -60), // Position above the button
child: Material(
elevation: 4.0,
child: Container(
padding: EdgeInsets.all(10),
child: Text('This is a tooltip positioned using LayerLink!'),
),
),
),
),
);
}
@override
void dispose() {
_overlayEntry?.remove();
super.dispose();
}
}
In this example, we use LayerLink
to position a tooltip directly above a button, regardless of where the button is on the screen. The tooltip is added to the overlay when the button is pressed and removed when pressed again.
Conclusion
LayerLink
is a powerful tool in Flutter for creating responsive and dynamically positioned UI elements. By allowing widgets to maintain their relative positions across different parts of the widget tree, it enables developers to create more complex and interactive user interfaces. Whether you’re working on tooltips, dropdown menus, or any other UI element that needs precise positioning, LayerLink
provides an efficient and flexible solution.
Remember to always dispose of your overlays properly to prevent memory leaks, and use LayerLink
judiciously to maintain clean and performant code. With practice, you’ll find LayerLink
to be an invaluable tool in your Flutter development toolkit.
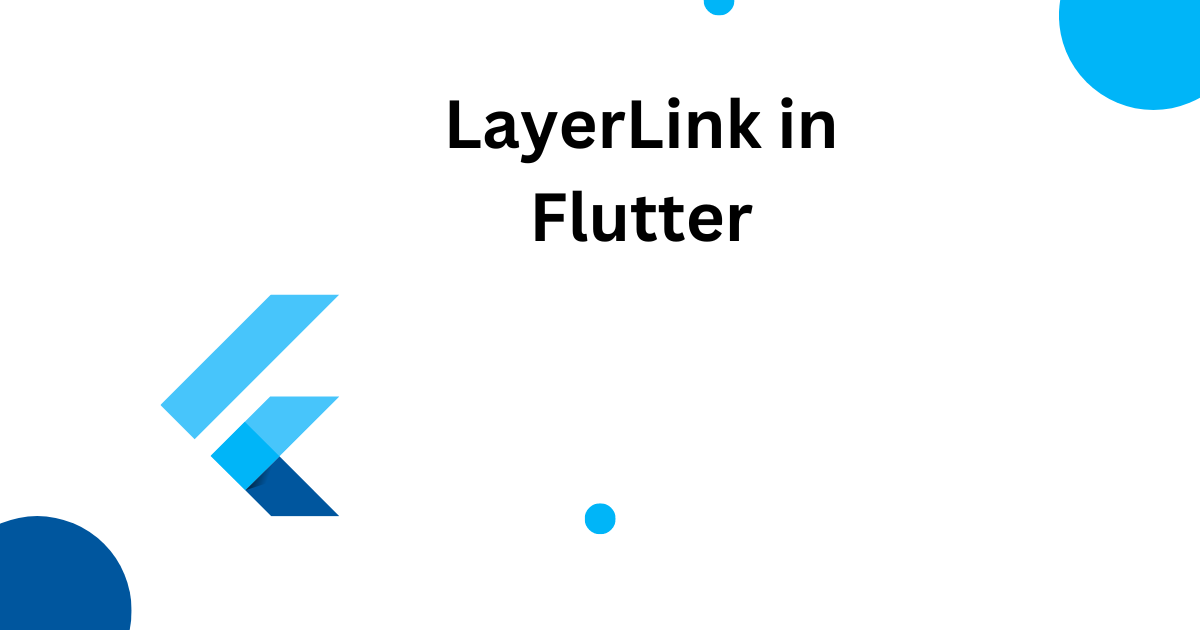
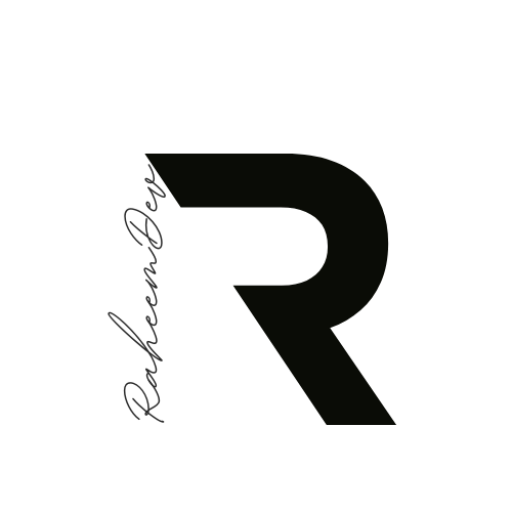