This article will guide you through the process of implementing Facebook account deletion in a Flutter app that uses Firebase authentication. This feature is essential for user privacy and compliance with data protection regulations.
Prerequisites
Before we start, ensure you have:
- A Flutter project set up
- Firebase integrated into your project
- Facebook Login implemented
Step-by-Step Guide
1. Add necessary dependencies
First, add the following dependencies to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
firebase_core: ^latest_version
firebase_auth: ^latest_version
flutter_facebook_auth: ^latest_version
Replace ^latest_version
with the actual latest versions of these packages.
2. Import required packages
In your Dart file, import the necessary packages:
import 'package:firebase_auth/firebase_auth.dart';
import 'package:flutter_facebook_auth/flutter_facebook_auth.dart';
3. Implement the account deletion function
Create a function to handle the Facebook account deletion process:
Future<void> deleteFacebookAccount() async {
try {
// Get the current user
final user = FirebaseAuth.instance.currentUser;
if (user == null) {
print('No user is currently signed in');
return;
}
// Re-authenticate the user
final LoginResult loginResult = await FacebookAuth.instance.login();
if (loginResult.status != LoginStatus.success) {
print('Facebook login failed');
return;
}
final AccessToken accessToken = loginResult.accessToken!;
final AuthCredential credential = FacebookAuthProvider.credential(accessToken.token);
await user.reauthenticateWithCredential(credential);
// Delete the user account
await user.delete();
// Log out from Facebook
await FacebookAuth.instance.logOut();
print('User account deleted successfully');
} catch (e) {
print('Error deleting user account: $e');
}
}
4. Call the deletion function
You can call this function when the user requests to delete their account, for example, by tapping a button:
ElevatedButton(
onPressed: deleteFacebookAccount,
child: Text('Delete Facebook Account'),
)
5. Handle post-deletion tasks
After deleting the account, make sure to:
- Clear any local storage or cache related to the user
- Navigate the user to a logged-out state or the app’s landing page
- Inform the user that their account has been successfully deleted
Important Considerations
-
User Confirmation: Always ask for user confirmation before initiating the account deletion process.
-
Data Deletion: Ensure that you delete all user data from your databases and storage, not just the authentication account.
-
Error Handling: Implement proper error handling to manage cases where the deletion process might fail.
-
Re-authentication: As shown in the code, re-authenticate the user before deletion. This is a security measure required by Firebase.
-
Facebook Developer Policies: Be aware of and comply with Facebook’s developer policies regarding data deletion and user privacy.
- Legal Compliance: Make sure your account deletion process complies with relevant data protection laws and regulations.
Conclusion
Implementing a Facebook account deletion feature in your Flutter app is crucial for user privacy and data protection compliance. By following this guide, you can provide your users with the ability to delete their Facebook-linked accounts within your app.
Remember to thoroughly test this feature and consider all edge cases to ensure a smooth user experience. Also, keep in mind that while this process removes the user’s account from your Firebase authentication, you may need to take additional steps to remove user data from Facebook’s systems, which typically involves actions on the user’s part directly with Facebook.
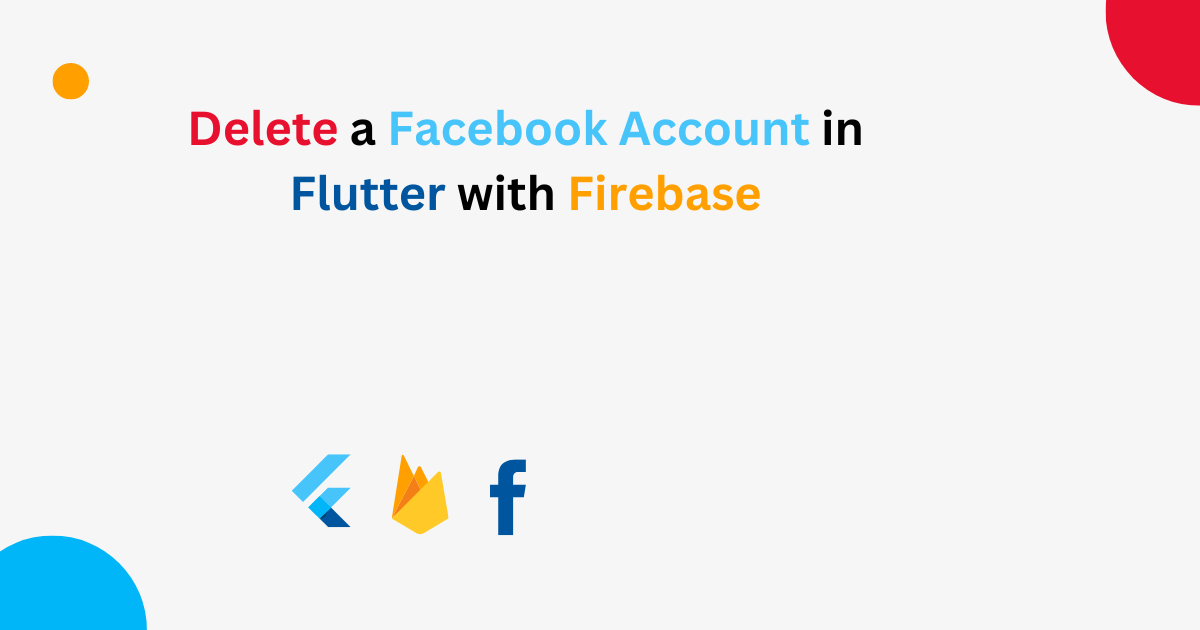
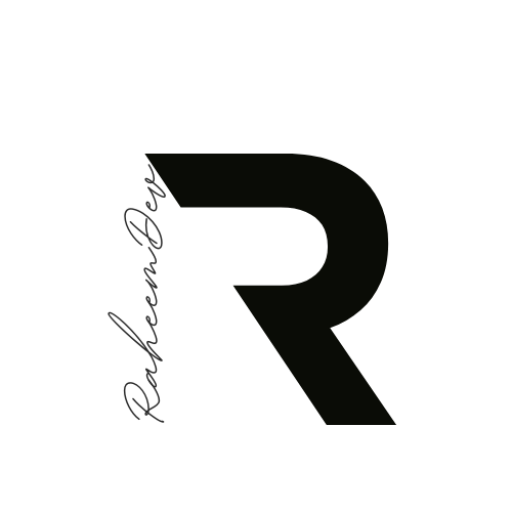